Created by N Padmavathi on Dec 29, 2023
Extension ID
com.castsoftware.jooq
What's new?
See jOOQ - 1.0 - Release Notes for more information.
Description
jOOQ (Java Object Oriented Query), is a library that generates Java classes based on database tables and allows the creation of type-safe SQL queries through its fluent API. This extension supports API for the jOOQ framework, which is responsible for the typical CRUD operations with the database.
In what situation should you install this extension?
If your Java application contains CRUD operations with fluent API or "raw" SQL queries in its source code provided by jOOQ packages and you want to view these object types and their links, then you should install this extension.
Technology support
Item | Version | Supported | Supported Technology |
---|
jOOQ | Up to : 3.18 |  | Java |
AIP Core compatibility
AIP Core release | Supported |
---|
8.3.x |  |
Supported DBMS servers
DBMS | Supported |
---|
CSS/PostgreSQL |  |
Download and installation instructions
For JEE applications using jOOQ, the extension will be automatically installed by CAST Console. This is in place since october 2023.
For upgrade, if the Extension Strategy is not set to Auto update, you can manually install the new release using the Application - Extensions interface.
What results can you expect?
Once the analysis/snapshot generation has completed, the following objects and links will be displayed in CAST Enlighten:
Objects
The following objects are displayed in CAST Enlighten:
Icon | Description | When is this object created ? |
---|
| JOOQ SQL Query | An object is created for each SQL query found and resolved in a jOOQ DSLContext method call. |
| JOOQ Entity | An object is created for each jOOQ Entity class found which inherits from Table TableImpl. |
| JOOQ Entity Operation | An object is created for each CRUD operation performed on Entity. |
Links
The following link types are created by the jOOQ extension, or by other extensions.
Link Type | Caller type | Callee type | Methods Supported |
---|
callLink | Java Method | jOOQ SQL Query | - org.jooq.DSLContext.fetch
- org.jooq.DSLContext.execute
- org.jooq.DSLContext.query
|
callLink | Java method | JOOQ Entity Operation | - org.jooq.ResultQuery.fetch
- org.jooq.ResultQuery.fetchOne
- org.jooq.ResultQuery.fetchAny
- org.jooq.ResultQuery.fetchLazy
- org.jooq.ResultQuery.fetchResultSet
- org.jooq.ResultQuery.fetchStream
- org.jooq.Query.execute
- org.jooq.Batch.execute
- org.jooq.ResultQuery.stream
- org.jooq.Query.executeAsync
|
useLink | jOOQ SQL Query | Table, View | Created by SQL Analyzer when DDL source files are analyzed |
callLink | jOOQ SQL Query | Procedure |
useLink | jOOQ Entity Operation | Table, View | Created by WBS when DDL source files are analyzed by SQL Analyzer |
callLink | jOOQ Entity Operation | Procedure |
useLink | jOOQ SQL Query | Missing Table | Created by Missing tables and procedures for JEE extension when the object is not analyzed. |
callLink | JDBC SQL Query | Missing Procedure |
Example code scenarios
Fluent API
Fluent API Examples
Select Operation
package com.Jooqexample.jooq;
import static com.JooQDemo.jooq.sample.model.Tables.*;
import com.JooQDemo.jooq.sample.model.tables.records.BikeRecord;
import com.JooQDemo.jooq.sample.model.tables.records.CarsRecord;
import org.jooq.*;
import org.jooq.Record;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.sql.ResultSet;
import java.util.stream.Stream;
@SpringBootTest
class SpringBootJooqApplicationTests {
@Autowired
private DSLContext dsl;
@Test
void DisplayData() {
CarsRecord Cars = dsl.selectFrom(CARS).fetchAny();
System.out.println("Result are -- " + Cars);
Result<Record> result0 = dsl.select().from(AIR_WAYS).fetch();
System.out.println("Result are1 -- " + result0);
Result<Record> result0 = dsl.select().from(CARS).stream();
Result<Record> result1 = dsl.select().from(CARS).fetch();
System.out.println("Result are1 -- " + result1);
Result<BikeRecord> result = dsl.selectFrom(BIKE).fetch();
System.out.println("Result are -- " + result);
ResultSet rs1 = dsl.selectFrom(CARS).fetchResultSet();
System.out.println("Result are -- " + rs1);
Cursor<Record1<String>> rs2 = dsl.select(CARS.MODEL_NAME).from(CARS).fetchLazy();
for (Record1<String> record : rs2){
System.out.println("Result are fetchlazy -- " + record);
}
Stream<Record1<String>> rs3 = dsl.select(CARS.MODEL_NAME).from(CARS).fetchStream();
rs3.forEach(record -> {
System.out.println("Result are fetchStream -- " + record);
// ...
});
CarsRecord Cars1 = dsl.selectFrom(CARS).where(CARS.MODEL_NAME.eq("Ertiga")).fetchOne();
System.out.println("Result are -- " + Cars1);
}
}
Example With Cars Entity
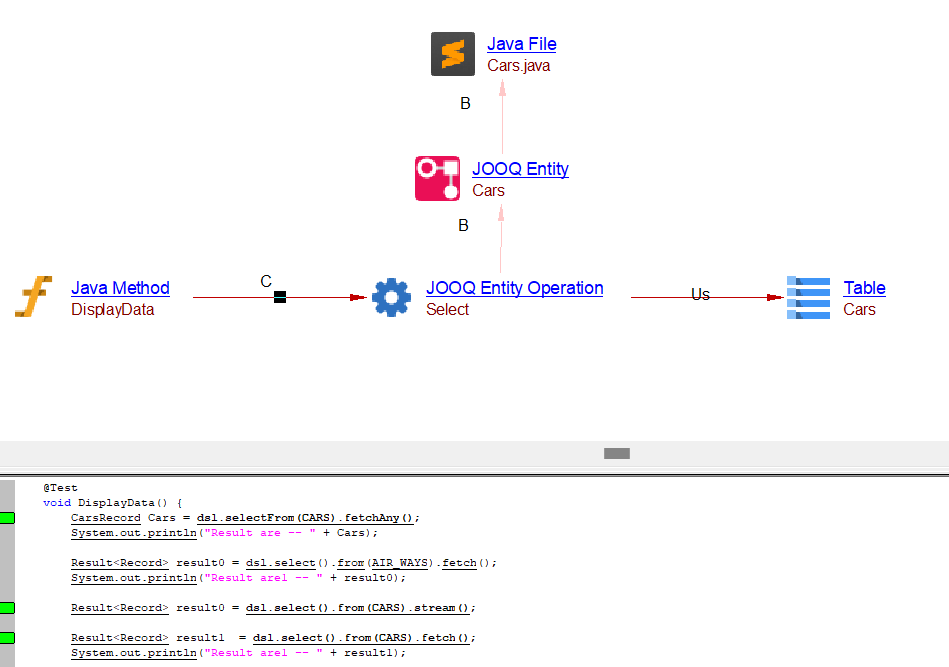
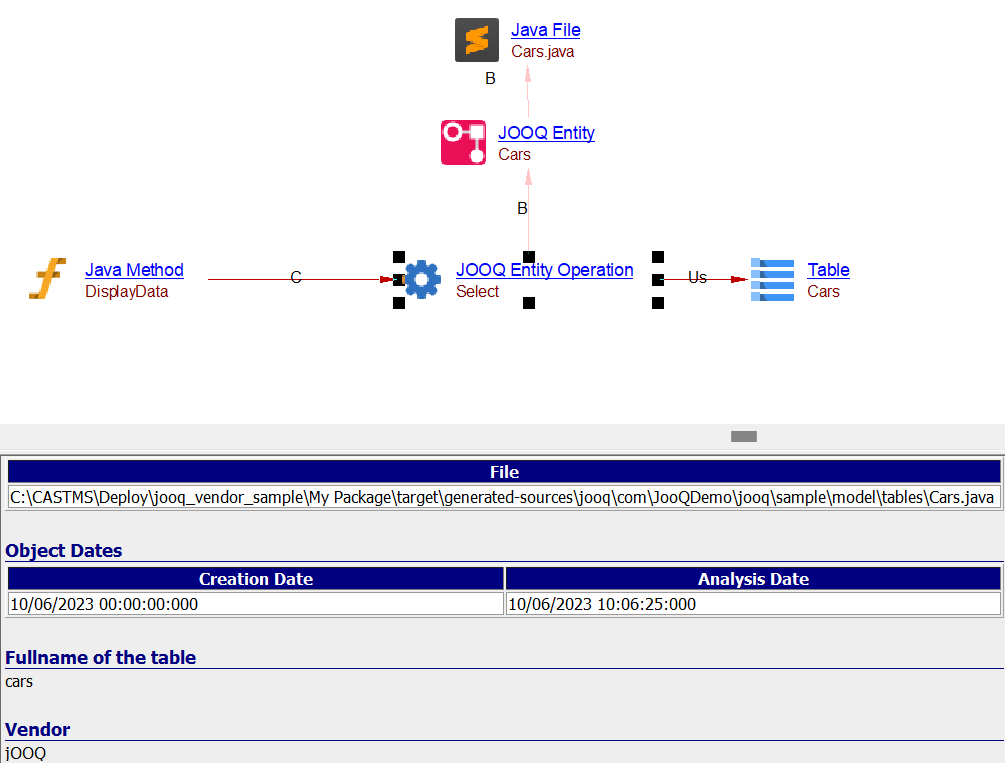
Example With Bike Entity
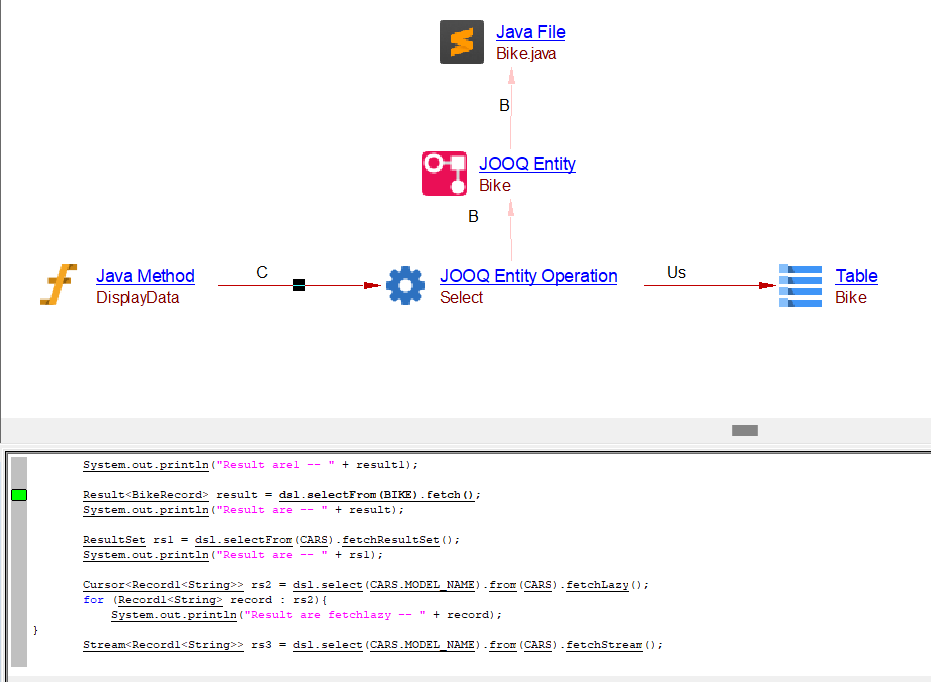
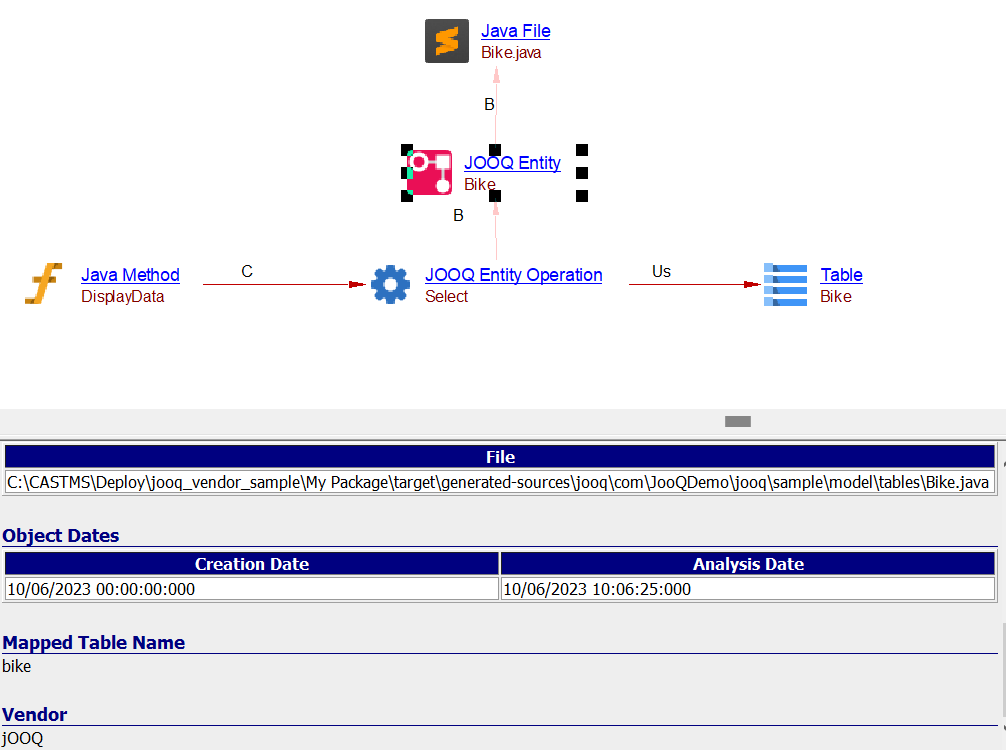
Insert Operation
void AddData() {
int insertedrow = dsl.insertInto(CARS).values(2,"TATA","NEXON",2012,100000000).execute();
System.out.println("Insert Operation Row Affected are -- " + insertedrow);
int insertedrow_bike = dsl.insertInto(BIKE).values(1,"Bajaj","Avenger",2012,150000).execute();
System.out.println("Insert Operation Row Affected are -- " + insertedrow_bike);
int insertedrow_bike2 = dsl.insertInto(BIKE).values(2,"Duke","KTM",2016,150000).execute();
System.out.println("Insert Operation Row Affected are -- " + insertedrow_bike2);
}
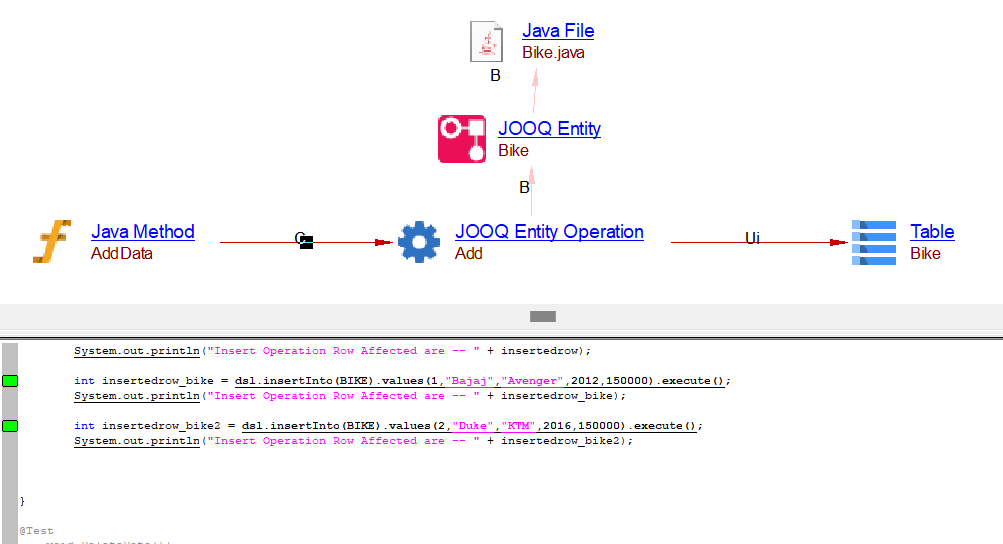
Delete Operation
void DeleteData(){
int deleterow = dsl.deleteFrom(CARS).where(CARS.ID.eq(2)).execute();
System.out.println("Delete Operation Row Affected are -- " + deleterow);
int deleterow_bike = dsl.deleteFrom(BIKE).where(BIKE.ID.eq(1)).execute();
System.out.println("Delete Operation Row Affected are -- " + deleterow_bike);
}
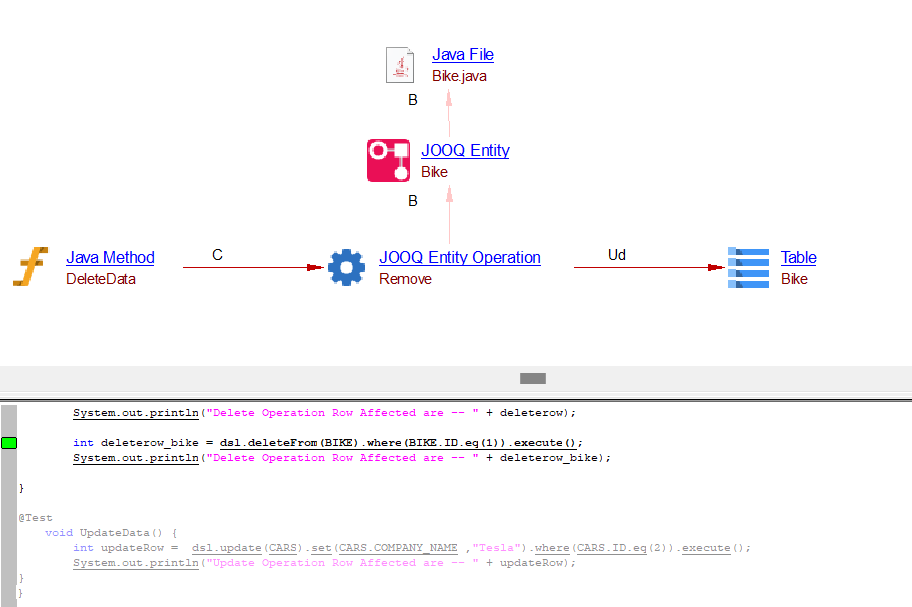
Update Operation
private void applyRuleFilter(HighlightDSLContext defaultDSLContext, Long aggregatedResultId, Long applicationId) {
defaultDSLContext.update(AGGREGATED_CLOUD_TECHNOLOGY)
.set(AGGREGATED_CLOUD_TECHNOLOGY.EXCLUSION, DSL.val(null,String.class))
.set(AGGREGATED_CLOUD_TECHNOLOGY.MALUSRATIO, AGGREGATED_CLOUD_TECHNOLOGY.EXCLUDED_MALUSRATIO)
.set(AGGREGATED_CLOUD_TECHNOLOGY.SCANINDICATOR, AGGREGATED_CLOUD_TECHNOLOGY.EXCLUDED_MALUSRATIO)
.set(AGGREGATED_CLOUD_TECHNOLOGY.COUNTEDELEMENTS, AGGREGATED_CLOUD_TECHNOLOGY.EXCLUDED_COUNTEDELEMENTS)
.where(AGGREGATED_CLOUD_TECHNOLOGY.AGGREGATED_RESULT_ID.eq(aggregatedResultId))
.and(AGGREGATED_CLOUD_TECHNOLOGY.EXCLUSION.isNotNull())
.execute();
}
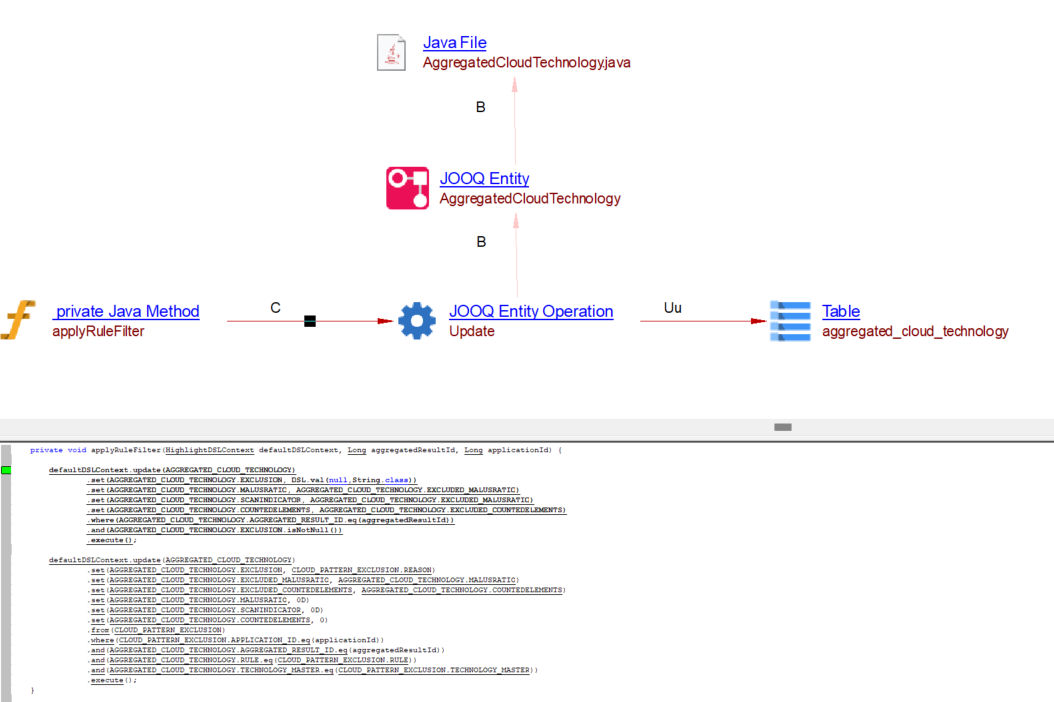
Batch Operation
void execute_batchUpdate() {
Result<BikeRecord> existingProjects = DSLContext.selectFrom(BIKE).where(BIKE.ID.eq(1));
DSLContext.batchUpdate(existingProjects.stream().collect(Collectors.toList())).execute();
}
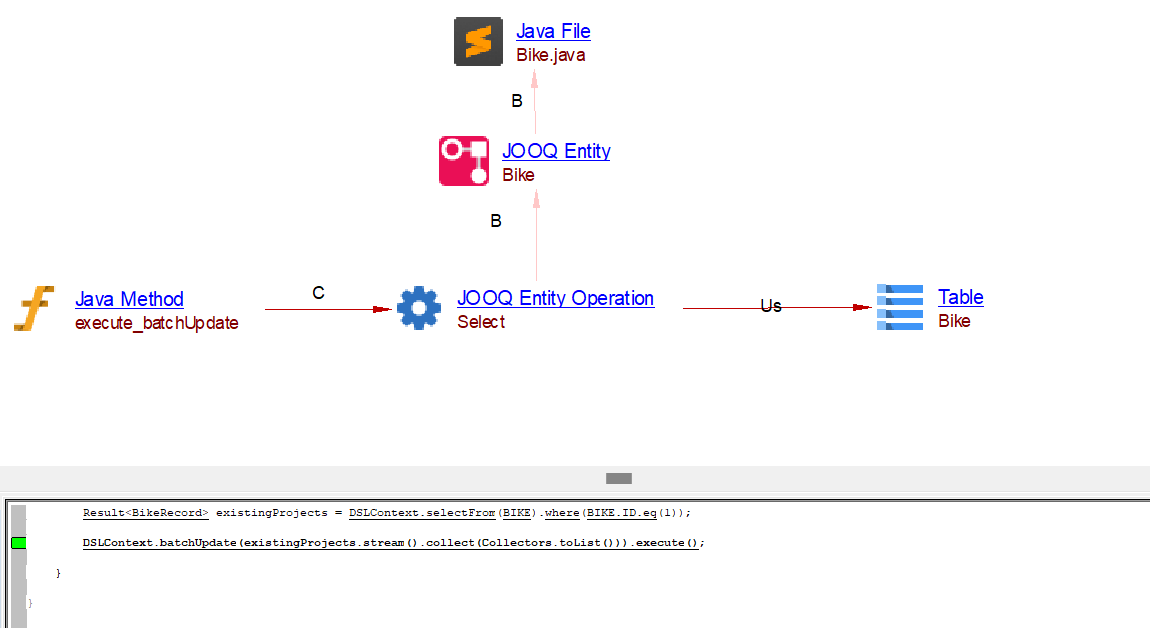
Raw SQL API
Raw SQL API Example
org.jooq.DSLContext.fetch
// Import the necessary jOOQ classes
import org.jooq.impl.DSL;
import org.jooq.*;
import org.jooq.Record;
import static org.jooq.impl.DSL.*;
public class JooqSample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/vehicle";
String uname = "root";
String password = "mysql";
String query = "select * from cars";
/////////// Creating the connection with database //////////
try {
DSLContext context = DSL.using(url, uname, password);
fetchRecord(context, query);
} catch (Exception e) {
System.out.println("\n Exception" + e);
}
}
public static void fetchRecord(DSLContext context, String query) {
try {
Result<Record> result = context.fetch(query);
System.out.println("Result are -- " + result);
} catch (Exception e) {
System.out.println("\n Exception " + e);
}
}
}
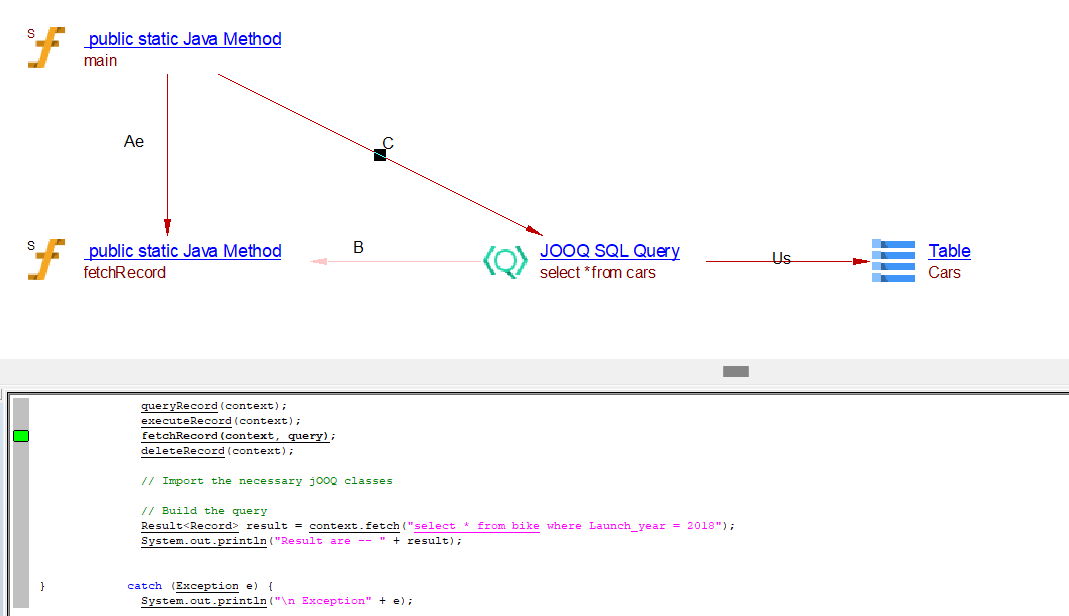
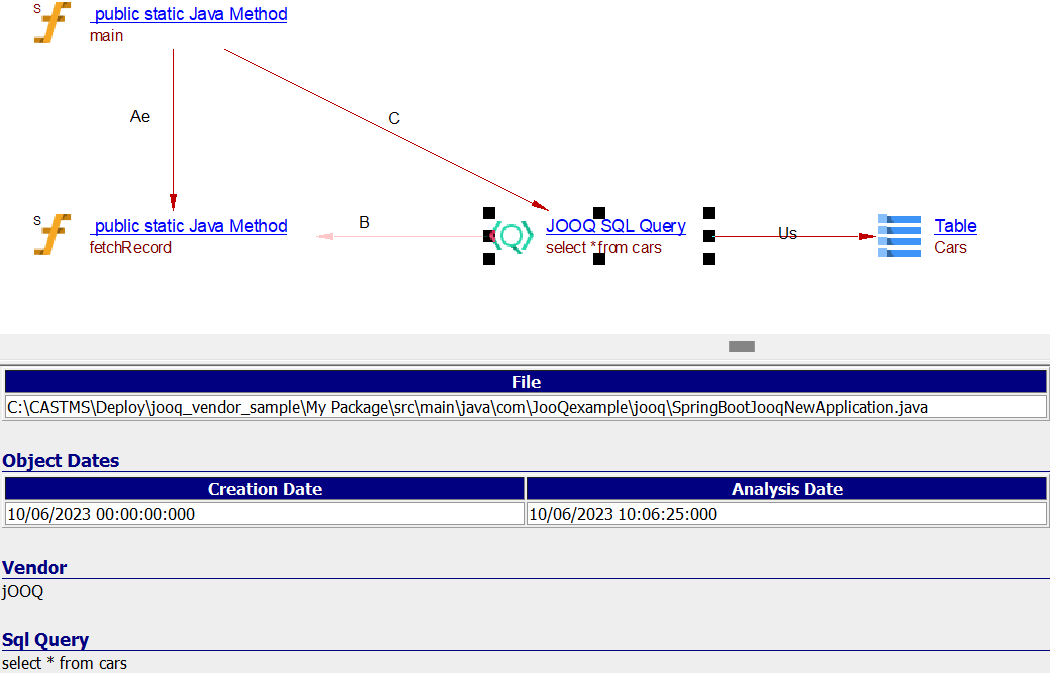
org.jooq.DSLContext.execute
public static void executeRecord(DSLContext context) {
try {
int rowCount = context.execute("insert into cars values(100,'Hyundai','verna',2016,1500000,3)");
System.out.println("Number of row affected are -- " + rowCount);
} catch (Exception e) {
System.out.println("\n Exception " + e);
}
}
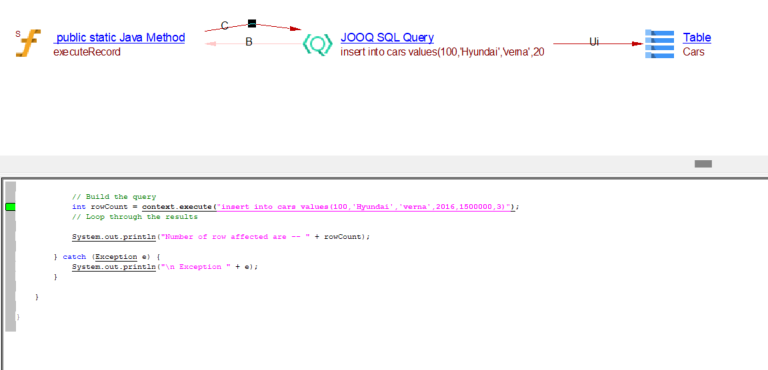
org.jooq.DSLContext.query
public static void queryRecord(DSLContext context) {
try {
Query query = context.query("insert into cars values(100,'Hyundai','verna',2016,1500000)");
System.out.println("Number of row affected are -- " + query);
} catch (Exception e) {
System.out.println("\n Exception " + e);
}
}
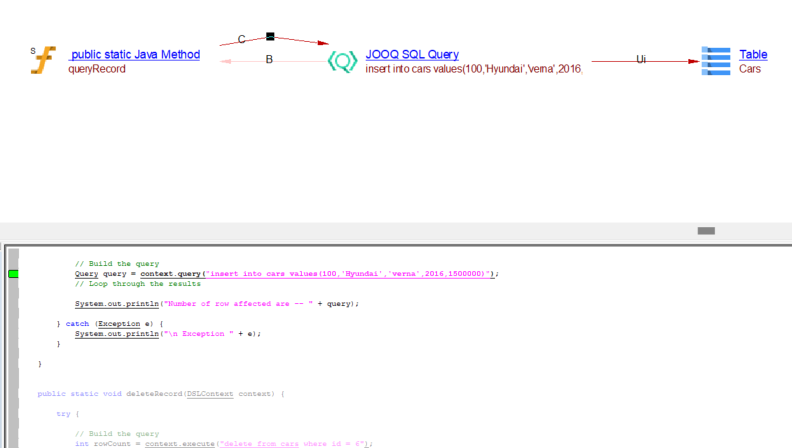
Limitations
- Objects will not be created if evaluation fails to resolve the necessary parameter.